class: center middle
# How to use pi camera with buttons and LED
<hr/>
## CyrusN
---
# Recipe
- Raspberry Pi
- Keyboard and mouse
- Power cable
- HDMI cable
- Ethernet cable
- Pi Camera
- Mini Breadboard
- LED light bulb
- 330 ohms resistor x 1
- Male to female dupont wires x 6
- Male to male dupont wires x 2
---
# Update and upgrade
Open Terminal app and enter the following commands
``` sh
sudo apt-get update
sudo apt-get upgrade
# Type yes if necessary
```
---
# Reference
- [gpiozero — Gpiozero 1.4.1 Documentation](https://gpiozero.readthedocs.io/en/stable/)
- [picamera — Picamera 1.13 Documentation](https://picamera.readthedocs.io/en/release-1.13/)
---
class: center
.img-100.scroll[

]
---
class: center middle
# Task 1: Connect a button
---
# Connect a button
.center.img-75[
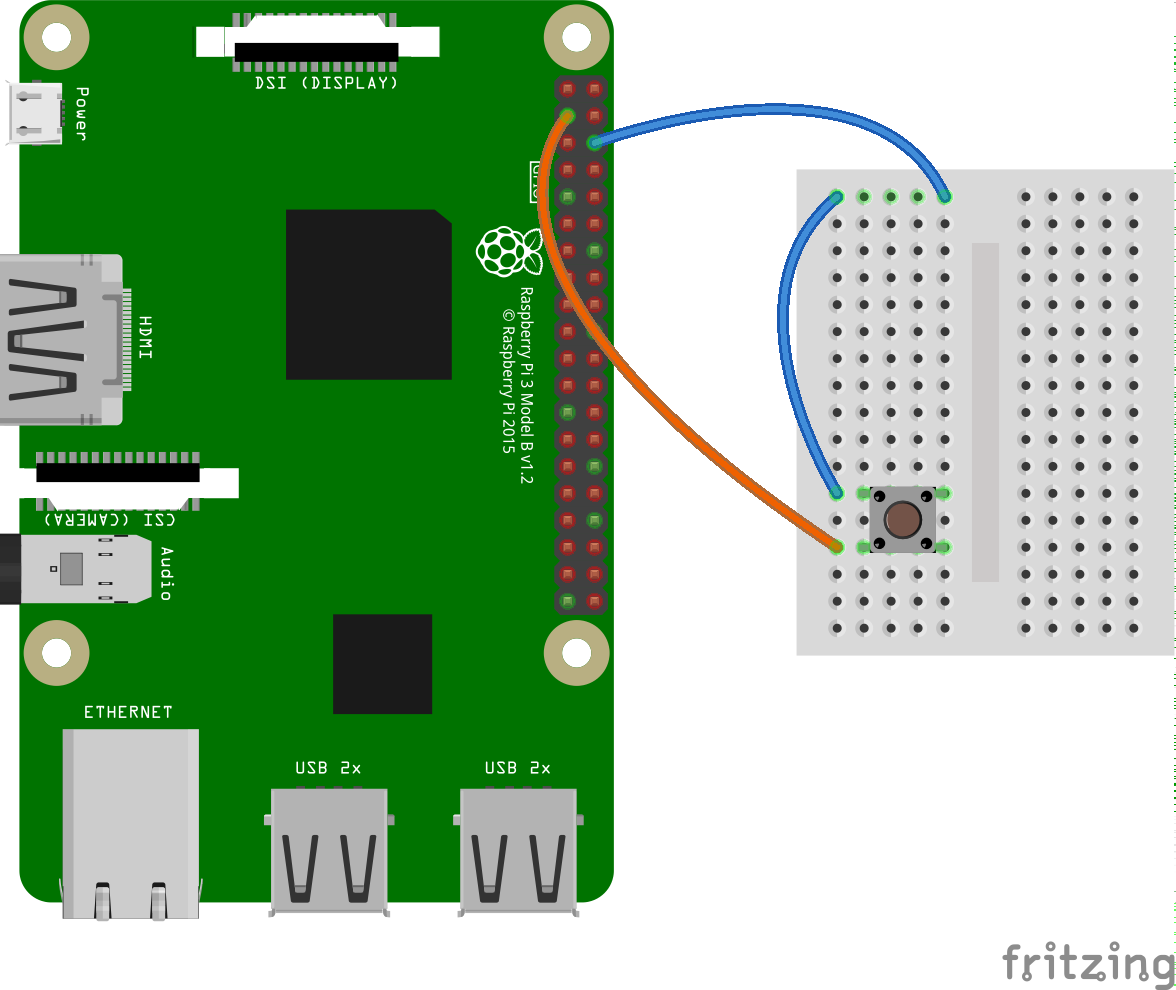
]
---
# Look inside a Button
.center.img-100[
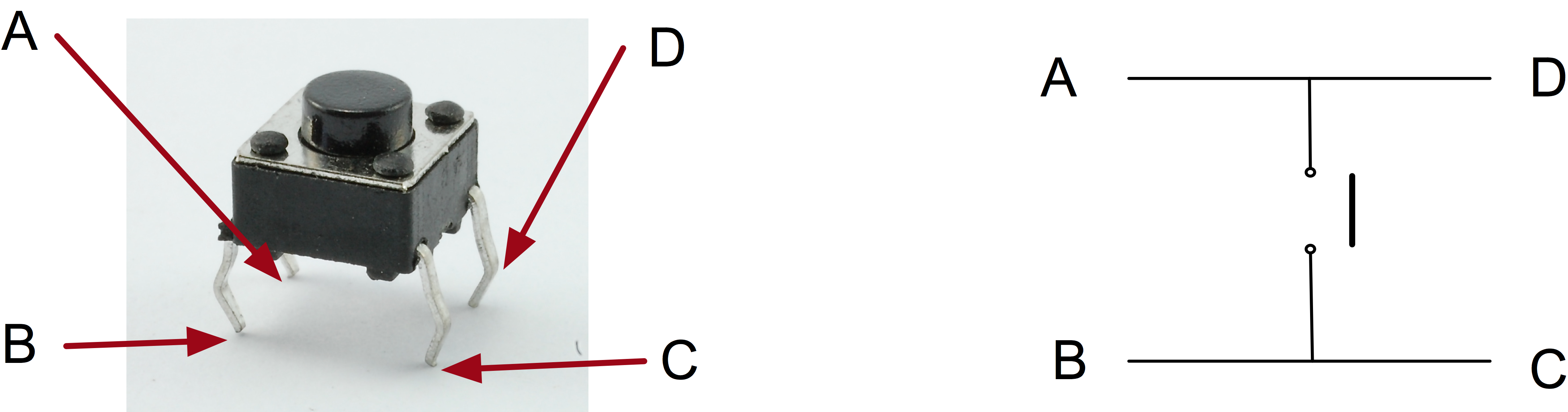
]
---
# Connect a button
- [`gpiozero.Button.when_pressed`](https://gpiozero.readthedocs.io/en/stable/api_input.html#gpiozero.Button.when_pressed)
- [How can I keep my script running](https://gpiozero.readthedocs.io/en/stable/faq.html#keep-your-script-running)
``` py
# import gpiozero and signal library
from gpiozero import Button
from signal import pause
button = Button(2)
# define a function call say_hello(), it simply prints the word "hello"
def say_hello():
print("Hello!")
# when button pressed, it invokes function say_hello()
button.when_pressed = say_hello
print("The Program is running")
pause()
```
---
class: center middle
# Copy the code
## Press F5 to run
---
class: center middle
# Control a LED with button
---
class: center middle img-75
# Control a LED with button
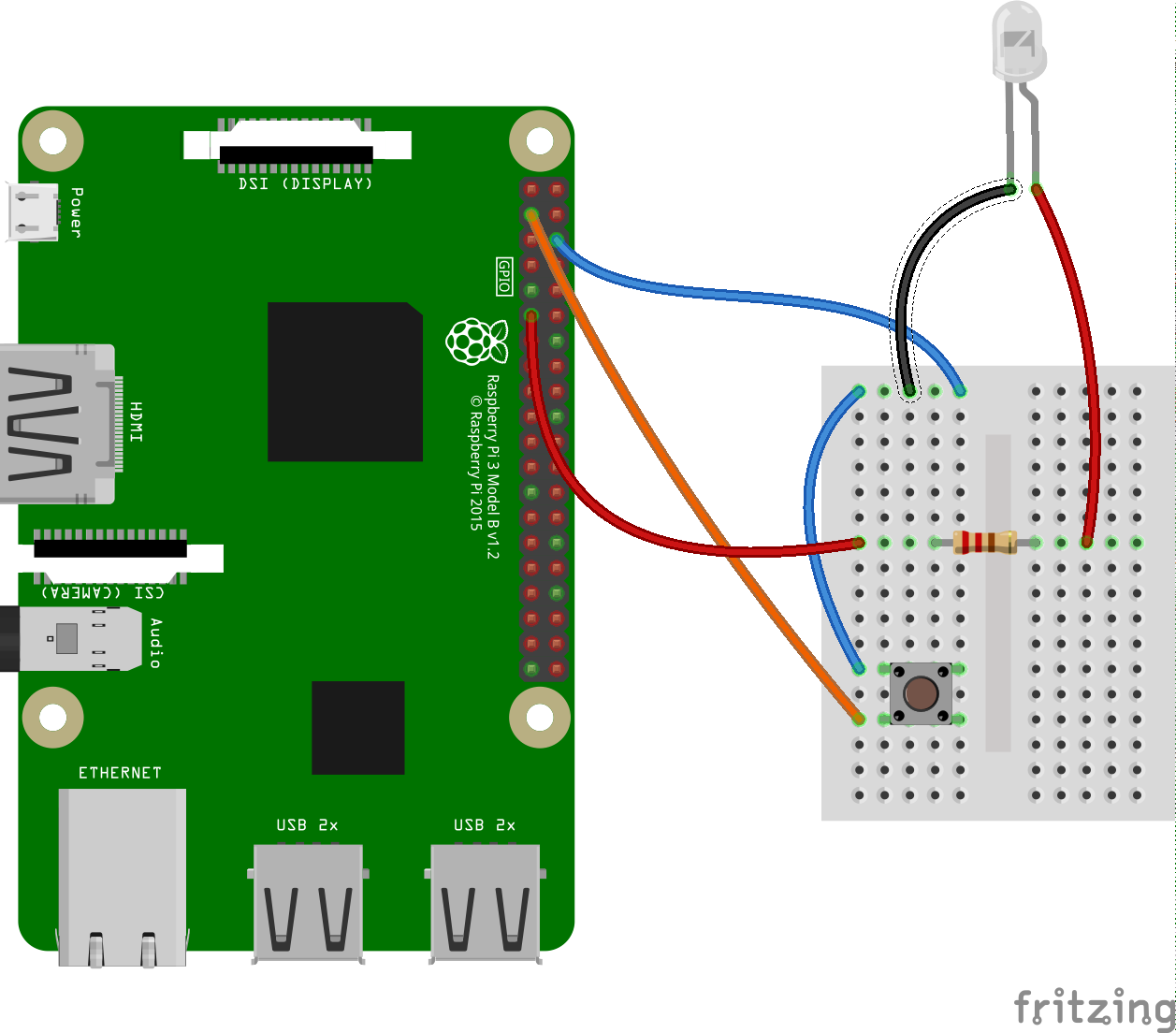
---
# Control a LED with button
[`gpiozero.LED.toggle`](https://gpiozero.readthedocs.io/en/stable/api_output.html#gpiozero.LED.toggle)
``` py
from gpiozero import LED, Button
from signal import pause
led = LED(17)
button = Button(2)
button.when_pressed = led.toggle
print("The Program is running")
pause()
```
---
class: center middle
# Copy the code
## Press F5 to run
---
class: center middle
# Connect to Pi camera
---
class: center middle img-75
# Setup
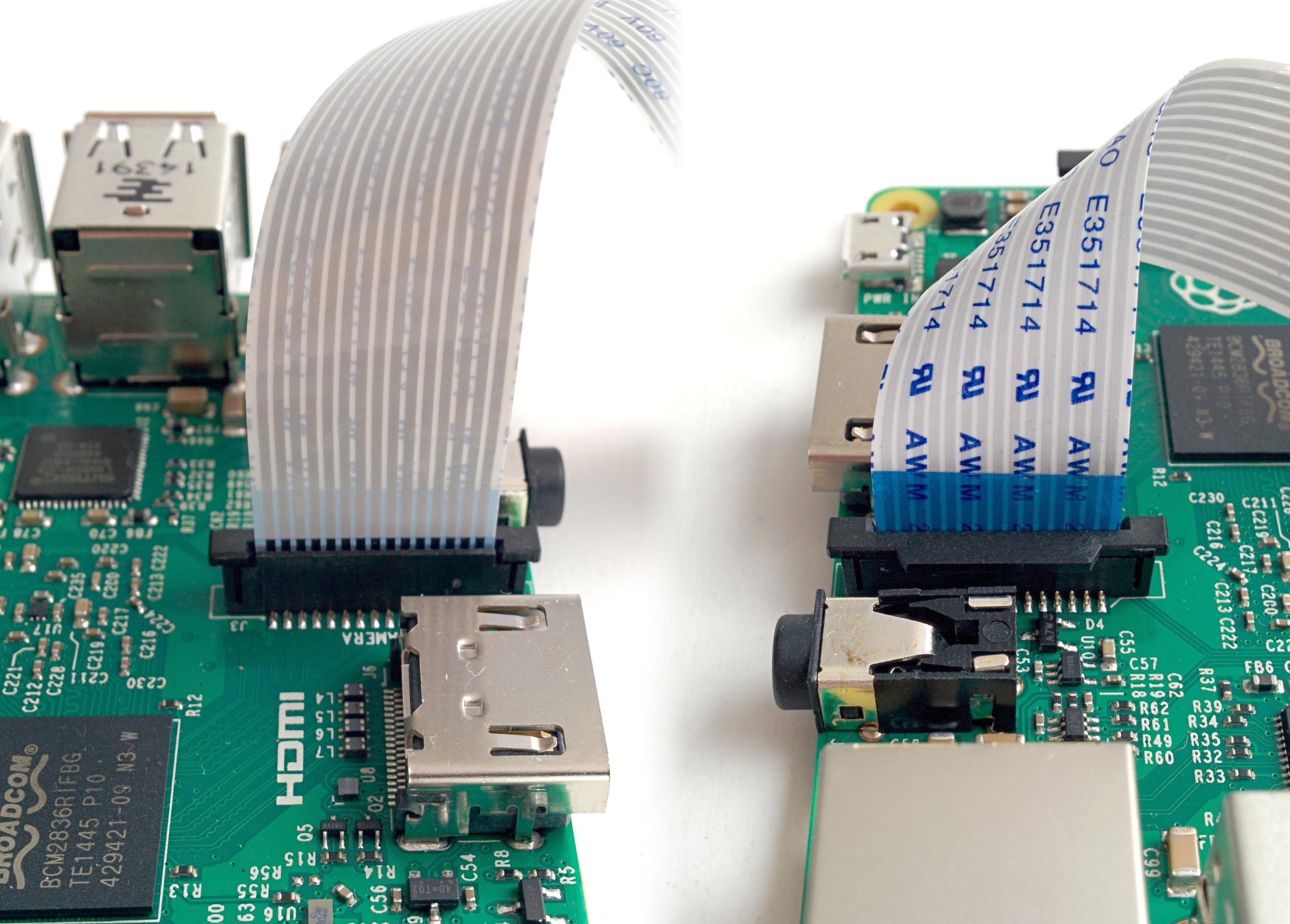
---
# Raspberry Pi Configuration
- Start the Raspberry Pi Configuration utility
- Enable the camera module
---
# Button control camera
- [`gpiozero.Button.when_released`](https://gpiozero.readthedocs.io/en/stable/api_input.html#gpiozero.Button.when_released)
- [`picamera.PiCamera.start_preview`](https://picamera.readthedocs.io/en/release-1.13/api_camera.html#picamera.PiCamera.start_preview)
- [`picamera.PiCamera.stop_preview`](https://picamera.readthedocs.io/en/release-1.13/api_camera.html#picamera.PiCamera.stop_preview)
```py
from gpiozero import Button
from picamera import PiCamera
from signal import pause
button = Button(2)
camera = PiCamera()
button.when_pressed = camera.start_preview
button.when_released = camera.stop_preview
print("The Program is running")
pause()
```
---
# Button to toggle preview and LED
- [picamera.PiCamera.previewing ](https://picamera.readthedocs.io/en/release-1.13/api_camera.html#picamera.PiCamera.previewing)
.scroll.img-100.h-75[
``` py
from gpiozero import Button, LED
from picamera import PiCamera
from signal import pause
button = Button(2)
led = LED(17)
camera = PiCamera()
def toggle_preview():
# toggle LED first
led.toggle()
# toggle preview, "camera.preview is None" means camera is not previewing.
if camera.preview is None:
camera.start_preview()
else:
camera.stop_preview()
print("The Program is running")
button.when_pressed = toggle_preview
pause()
```
]
---
class: center middle
# Copy the code
## Press F5 to run
---
class: center middle
# Capture image with button
---
# Capture image with button
- [`datetime.now`](https://docs.python.org/3.7/library/datetime.html#datetime.datetime.now)
- [`datetime.isoformat`](https://docs.python.org/3.7/library/datetime.html#datetime.datetime.isoformat)
- [`os.path.join`](https://docs.python.org/3.7/library/os.path.html#os.path.join)
- [`PiCamera.capture`](https://picamera.readthedocs.io/en/release-1.13/api_camera.html#picamera.PiCamera.capture)
---
# Capture image with button
```py
from gpiozero import Button
from picamera import PiCamera
from datetime import datetime
from os import path
from signal import pause
button = Button(2)
camera = PiCamera()
CAPTURE_DIR = "/home/pi/Desktop"
def capture():
now = datetime.now().isoformat()
filename = path.join(CAPTURE_DIR, now + ".jpg")
camera.capture(filename)
print("Image Captured: ", filename)
button.when_pressed = capture
print("The Program is running")
pause()
```
---
class: center middle
# Connect 1 more button on pin 3
---
# Bring all together
- set pin 2 as left button
- use left button to toggle preview
- LED is only on when previewing,
- set pin 3 as right button
- use right button to capture image
- image can only be captured while previewing
---
class: center middle
# Bring all together
.img-50[
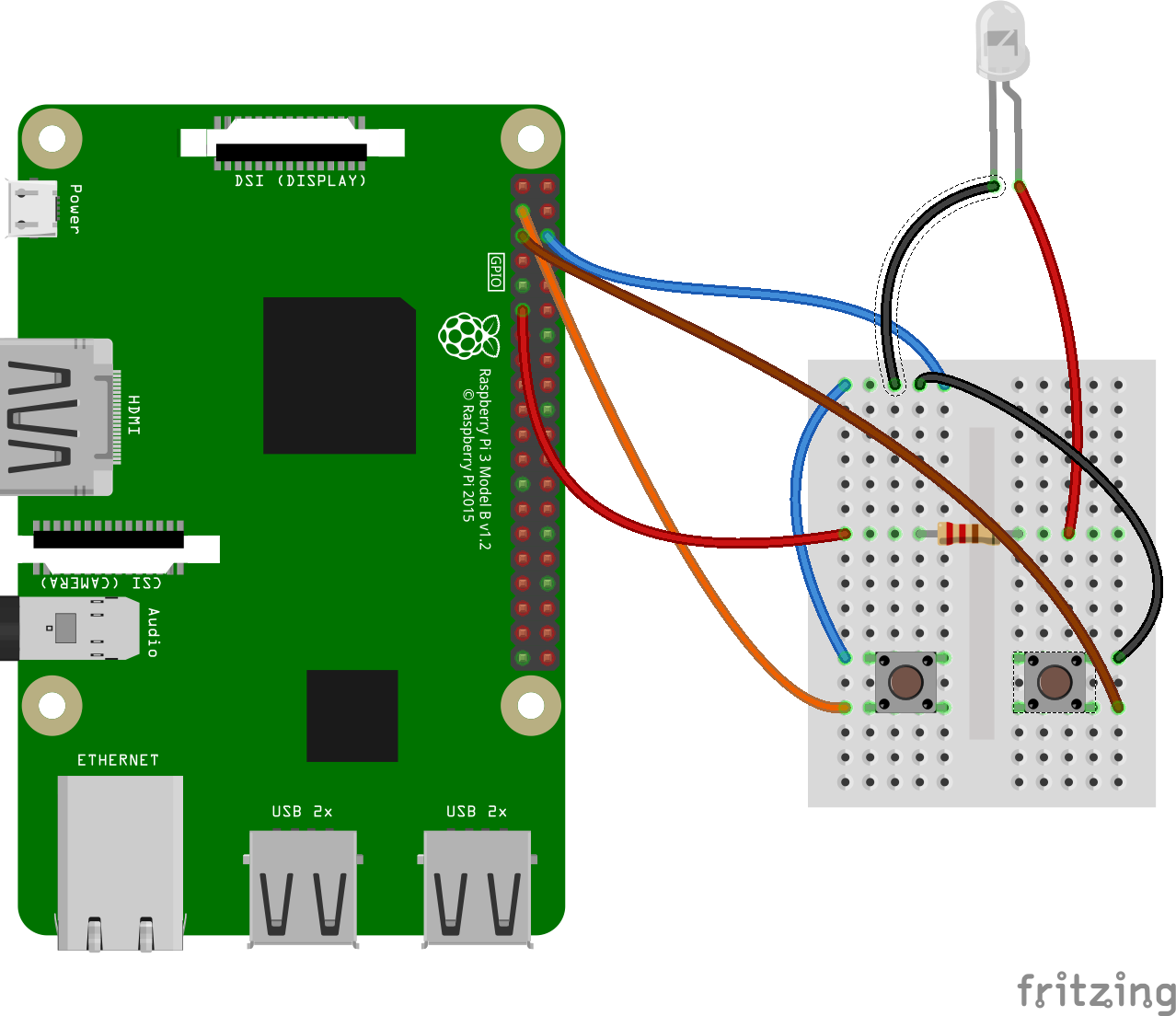
]
---
# Bring all together
.scroll.h-75.img-100[
``` py
from gpiozero import Button, LED
from picamera import PiCamera
from datetime import datetime
from os import path
from signal import pause
left_button = Button(2)
right_button = Button(3)
led = LED(17)
camera = PiCamera()
CAPTURE_DIR = "/home/pi/Desktop"
def capture():
if camera.preview is not None:
now = datetime.now().isoformat()
filename = path.join(CAPTURE_DIR, now + ".jpg")
camera.capture(filename)
print("Image Captured: ", filename)
def toggle_preview():
led.toggle()
if camera.preview is None:
camera.start_preview()
else:
camera.stop_preview()
left_button.when_pressed = toggle_preview
right_button.when_pressed = capture
print("The Program is running")
pause()
```
]
---
class: center middle
# Copy the code
## Press F5 to run
---
class: center middle
# .text-danger[♥] Thank you .text-danger[♥]